I. Introduction
II. Starting a new project
III. The structure of a project
IV. Adding your own sources and applications
V. Creating a new package or how to fit your code into the Black Box philosophy
VI. System architecture and further info
I. Introduction
After installing the CreaTools you will have direct acces to several tools like the bbEditor, bbi and creaNewProject, that you will use according to your needs as a script, package or library developer. Below you can find a non-exhaustive list of the available tools:
1 - Tools for Script (bbg/bbs) Developers
- 1.1- bbEditor
- 1.2- bbStudio
- 1.3- bbi
2 - Tools for Package and library (C++) Developers
- 2.1- CreaNewProject
- 2.2- Create Package
- 2.3- Create black box
II. Starting a new project
If you want to develop a new (your own) package or library, you should begin by creating a new project inside which you can place your packages and libraries. The main steps for creating a new project are:
1. Create your project using the CreaTools interface
- Use creaTools -> DevelTools -> C++ -> CreaNewProject
- Inside your new project, a new package is created automatically. You can also create other packages, new libraries or applications. New BlackBoxes are to be created into one of your packages.
2. Configure your project using CMake
- Create a project_bin folder outside (at the same level as) your project folder.
- Run CMake on the CMakeLists.txt file at the base of your project folder.
- Select (turn ON) all packages you want to compile.
- Note that you need to have installed the optional libraries available on the download page.
3. Compile your project using an appropriate compiler (e.g. gcc on Linux or Visual C++ on Windows)
- Using Visual C++ on Windows: open the newly created .sln project (in your bin folder) and build solution using RelWithDebInfo
- Using gcc on Linux:
4. Add your project as a BBTK plugin
- Note that you need to have built (at least) one package inside your project (turn it ON at the configuration phase).
- Use creaTools -> DevelTools -> bbStudio -> tools -> Plug Package.
- Select the bin folder of your project (that contains one or more packages)
- Close bbStudio and bbEditor.
Here's a summary of the main steps to follow for creating your new project:
1- CreateNewProject
|
creaTools -> DevelTools -> C++ -> CreaNewProject
=> (create in <PathDirPrj><MyProject>) ...more details here ...
|
1.1- Create a new Library
|
<MyProject>/lib
|
1.2- Create a new Application (optional)
|
<MyProject>/appli
|
1.3- Create a Package BBTK
(Automatic the first time)
|
For the first package this step is automatically.
See bbtk_<Myproject>_Package.
creaTools -> DevelTools -> C++ -> CreatePackage
=> (put your result files in <MyProject>)
|
1.3.1- Create a black box BBTK
|
creaTools -> DevelTools -> C++ -> CreateBox
=> (put your result files in <MyProject><YourPackage>/src)
|
2- Compile the newProject
|
Use CMAKE to configure your project. Create
|
3- Install the newProject (optional)
|
Define the Install-Prefix of your project in the CMake-Configuration
=> <PathDirPrj-Install>
|
4- Add newProject as a plugin of BBTK
|
creaTools -> DevelTools -> bbStudio -> tools -> AddPlugin.
|
III. The structure of a project
- CMakeLists.txt - the root CMakeLists.txt file is needed for the project configuration. You can use any of the libraries listed in the automatically generated file by turning them "ON". For exemple, to compile packages you need to set USE_BOOST to ON "SET(USE_WXWIDGETS ON)". Also note that to compile new packages that have not been automatically generated, you need to include the corresponding directory in CMakefiles.txt : "SUBDIRS(
) " - lib - the lib folder contains a CMakeLists.txt configuration file and your library folders (containing your src.cxx and src.h files). See also the next section IV. Adding your own sources and applications
- appli - the appli folder contains a CMakeLists.txt configuration file and your application folders (containing your main.cxx files). See also the next section IV. Adding your own sources and applications
- bbtk_mySamplePackage_PKG - the package folder contains
- CMakeLists.txt - a CMakeLists.txt package configuration file similar to the project one
- src - the src folder containing the sources of your "atomic" black boxes, which are cxx/h files (may be automatically generated from their XML description).
- bbs - the Black Box Scripts folder containing the appli and boxes folders. Both contain your .bbs files written using the bb scripting language, which allows you to create pipelines chaining existing boxes
IV. Adding your own sources and applications
You may already have your own developements that you want to integrate into the newly created project. In that case, you should add your main files into the appli folder and your other sources into a new folder inside the automtically generated lib folder. If your sources can be structured into multiple libraries, create one new folder for each of them inside the main lib folder. You can find below further instructions for creating and compiling your libraries and applications inside your new CreaTools Project.
1. Creating a new library
To add a new library go to the lib directory of your project (e.g. /Creatis/creaExample/lib)- Create a new directory and name it according to the library you want to create (e.g. /Creatis/creaExample/lib/exampleLib)
- Edit the CMakeLists.txt in the lib folder (/Creatis/creaExample/lib/CMakeLists.txt) by adding a SUBDIRS() command (e.g. SUBDIRS(exampleLib))
- Copy the CmakeLists.txt file from the template lib folder into the directory of the library you want to create (e.g. copy /Creatis/creaExample/lib/template_lib/CmakeLists.txt into /Creatis/creaExample/lib/exampleLib)
- Edit the copied CMakeLists.txt by changing the library name (e.g. change SET(LIBRARY_NAME MyLib) to SET(LIBRARY_NAME exampleLib) )
- If this library must link against other libraries, add them or uncomment the lines in the SET ( ${LIBRARY_NAME}_LINK_LIBRARIES option (e.g. if you need to link against VTK, remove the "#" before ${VTK_LIBRARIES})
- Add your source files into your lib directory (e.g. exampleLib)
-
Note: The default is to create a DynamicLibrary, if you need to create a static library,
- Put
_BUILD_SHARED OFF in CMake OR - comment the line CREA_ADD_LIBRARY( ${LIBRARY_NAME} ) in line 58, and add the following linesADD_LIBRARY(${LIBRARY_NAME} STATIC ${${LIBRARY_NAME}_SOURCES})TARGET_LINK_LIBRARIES(${LIBRARY_NAME} ${${LIBRARY_NAME}_LINK_LIBRARIES} )
- Put
- Add to the /lib/CmakeLists.txt file the directory of your new library : subdirs(exampleLib)
2. Creating the executable for your application
To add a new application go to the appli directory of your project (e.g. /Creatis/creaExample/appli)- Create a new directory according to the name of the application (e.g. /Creatis/creaExample/appli/exampleAppli)
- Edit the CMakeLists.txt in the appli folder (e.g. /Creatis/creaExample/appli/CMakeLists.txt) by adding a SUBDIRS() command (ex. SUBDIRS(exampleAppli))
- Copy the CmakeLists.txt file from "/appli/template_appli/CmakeLists.txt" into the directory of the application you want to create (e.g. copy /Creatis/creaExample/appli/template_appli/CmakeLists.txt into /Creatis/creaExample/appli/exampleAppli)
- Edit the copied CMakeLists.txt by changing the EXE_NAME in the top (e.g. change SET(EXE_NAME MyExe) to SET(EXE_NAME exampleAppli))
-
If the exe file links against other libraries:
- add the path of the .h files in line INCLUDE_DIRECTORIES ex. ../../lib/
- add or uncomment the lines in the SET ( ${LIBRARY_NAME}_LINK_LIBRARIES option (e.g. uncomment ${WXWIDGETS_LIBRARIES}, ${VTK_LIBRARIES} and add exampleLib (library created in step 2))
- add the path of the .h files in line INCLUDE_DIRECTORIES ex. ../../lib/
V. Creating a new package or how to fit your code into the Black Box philosophy
Now that you have included your sources into a CreaTools project, you may want to use them as BlackBoxes and integrate them as components of .bbs scripts. In order to do so, you need to create a package inside your project (e.g. /Creatis/creaExample/MyPackage). The sources of your Atomic Black Boxes are to be placed/found inside the src folder of the corresponding package (e.g. /Creatis/creaExample/MyPackage/src). Your pipelines or Complex Black Boxes (bbs scripts) are to be placed/found inside the bbs folder of the corresponding package (e.g. /Creatis/creaExample/MyPackage/bbs) In order to be able to use your libraries (the one you have just added to your project), you need to include them in your package. To do so you have to modify the CMakefile.txt of your PACKAGE as follows:
VI. System architecture and further info
More detailed documentation can be found here
Below you can find a simplified view of the CreaTools' architecture.
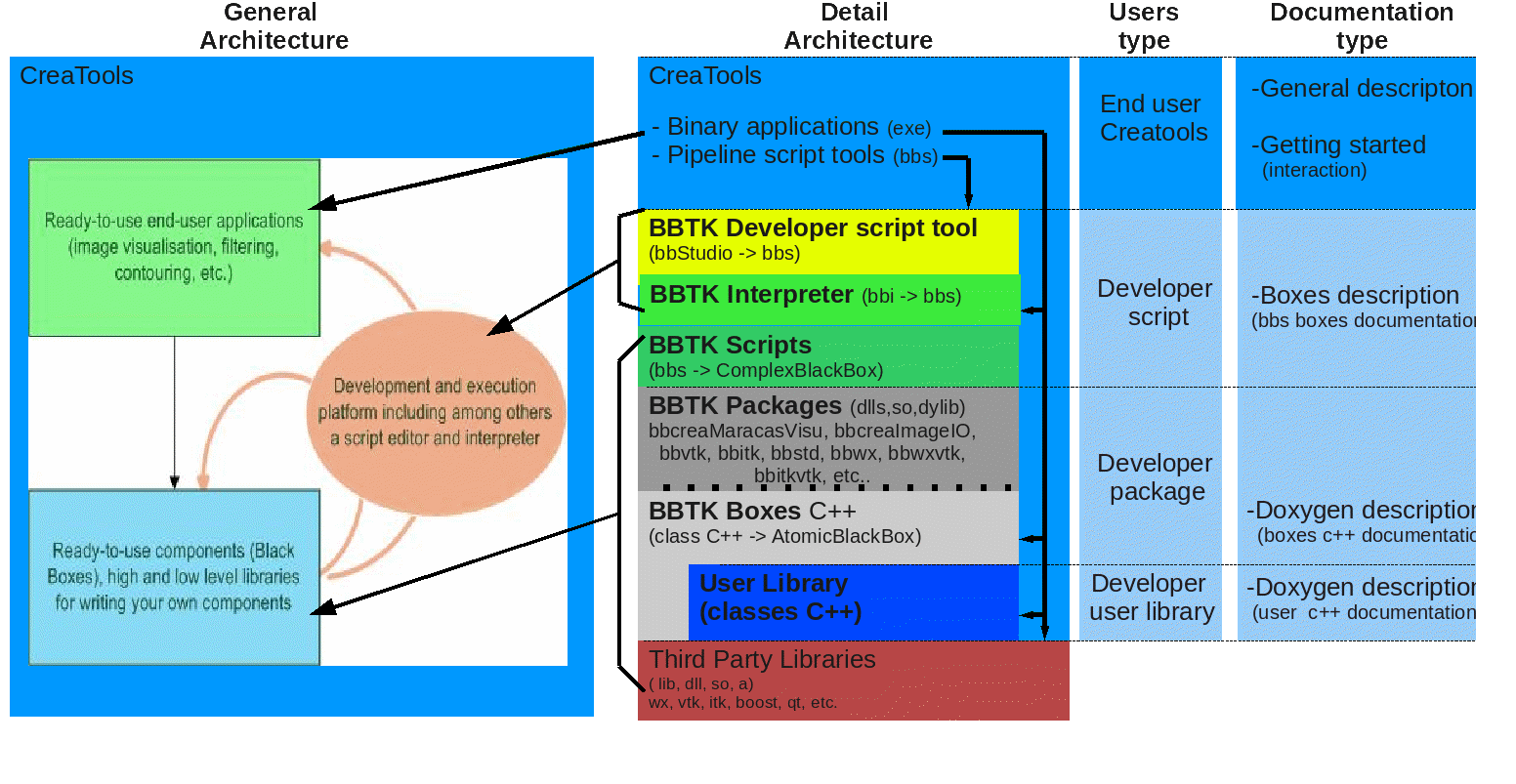